JWT authentication with Delphi. Part 1
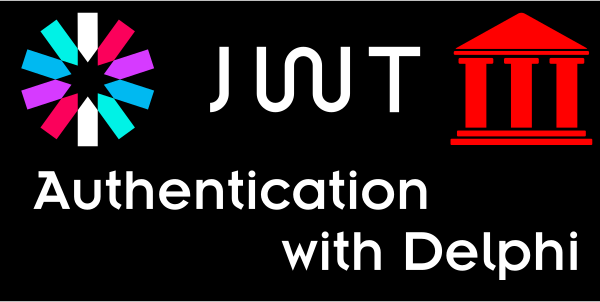
JWT Authentication with Delphi Serie
- Part 1: Authorization and JWT basic concepts
- Part 2: The JWT in depth
- Part 3: Building and verifying JWTs in Delphi
- Part 4: Using the Consumer to validate the JWT
This is the first article I will write about JWT and authentication technologies using Delphi, specifically I'll cover the topic of authentication (mostly in a HTTP world) using tokens (specifically JSON Web Tokens).
The Delphi library used in this article is the open source delphi-jose-jwt library (created by me) and available on GitHub. This library is listed on the JWT.io site and it's already used in several projects (open source and commercial) but before we dive into the code let’s cover some basics about JWT's.
Authentication
Authentication is the process of identifying someone (or something) determining that is who is claimed to be. Remember that in this article I will be speaking only about authentication and not authorization (that is giving individuals access to system objects based on their identity)
Session based authentication
In the HTTP world, applications have traditionally used session cookies to store authentication information. The mechanism relies on session IDs stored server-side. The session storage is typically an in-memory list/table (that is server-specific), or a separate session storage layer (often the back-end database).
In HTTP the communication is essentially stateless, so the cookie (that contains the session ID) is used by the server as a “key” to retrieve information about the client side (user or process). The cookie is created by the server and sent to the client, in the next request, the cookie is bounced back so the server can lookup the session ID in the session table.
Disadvantages of “cookie” based auth:
- Sessions: Sessions are just stored on server’s memory
- Mobile: Native mobile apps seems to have problems working with cookies so if we need to query a remote API, maybe session auth is not the best solution.
- CSRF: (Cross-Site Request Forgery) If we go down the cookies way, you really need to do CSRF to avoid cross site requests. That is something we can forget when using JWT as you will see.
- CORS: (Cross-Origin Resource Sharing) Have you fight with CORS and cookies? No need to wrestle using JWT.
Token based authentication
The main problem of a session based authentication is that the server must maintains a list of session to be able to “validate” the incoming request and that is a problem because only one server knows how to validate a client request (no scalability or availability).
So, token authentication was developed to solve problems of server-side session IDs. Using tokens instead of session IDs has the effect of lowering the server load and remove the need of storing an in-memory session table or having an expensive session storage layer (performance). JWT are stateless by definition so they are perfect for this task (more on that, later).
Before a token is created the user must, obviously, supply verifiable credentials (standard username/password pair, API keys, hardware IDs, or even tokens from another service) and consequently perform some sort of “login” action.
Keep in mind that JWT is not the only “standard” token representation out there, SWT (Simple Web Token) is (was) a proposed standard (Microsoft 2009) and SAML (Security Assertion Markup Language Token) is an open-standard for exchanging authentication and authorization data between parties based on XML (SAML 2.0, OASIS Standard 2005).
OAuth 2
Often I read about OAuth 2 and JWT as if they were comparable (and competing) standards… well, they are not!
OAuth 2 is an authorization framework that can employ JWT as the format for the OAuth 2 tokens, remember that OAuth2 is not an authentication protocol (because OAuth2 doesn’t know nothing about the user). OAuth 2 is a rather complex topic and I think I will write another article on this topic.
JSON Web Token (JWT)
A JSON Web Token or JWT (pronounced “jot”) is a signed piece of data in JSON format and because it's signed the recipient (the server) can, and must, verify its authenticity.
The workflow is basically this: a user wants to authenticate so he sends the username and password (for example), the server validates the user and creates a (cryptographically) signed token and then sends it back to the user. The user sends the token with the next request and the server checks if the signature is genuine and (eventually) grants the access to the requested resource. In detail:
- The user sends username and password to an authentication service
- The authentication service responds with a signed JWT with information about the user
- The user requests a resource on the server sending the token back
- The server checks the signature and if it's genuine the access is granted
A first look to a JWT
This is what a JWT looks like:
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiYWRtaW4iOnRydWV9.eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9
The same JWT decoded:
{
"alg": "HS256",
"typ": "JWT"
}
.
{
"sub": "1234567890",
"name": "John Doe",
"admin": true
}
.
HMACSHA256(
base64UrlEncode(header),
base64UrlEncode(payload),
secret
)
As you can see the token is composed of three parts:
- In the first part (called header) are stored information about the signing algorithm and the type of payload (JWT)
- The second (the payload) contains the actual user data (claims)
- The third part is the signature computed (in this example) with the HMACSHA256 algorithm.
What is JOSE?
Before I talked about the Delphi JWT library delphi-jose-jwt, but what is JOSE? (and no, is not my name!). JOSE stands for JSON Object Signing and Encryption and is a (set of) standard that provides a general approach to signing and encryption of any content. JOSE consists of several RFC:
- JWT (JSON Web Token) describes representation of claims encoded in JSON
- JWS (JSON Web Signature) describes producing and handling signed messages
- JWE (JSON Web Encryption) describes producing and handling encrypted messages
- JWA (JSON Web Algorithms) describes cryptographic algorithms used in JOSE
- JWK (JSON Web Key) describes format and handling of cryptographic keys in JOSE
In a nutshell: the JWT contains the claims, the JWS is the JWT when signed, the JWE is the JWT when encrypted, the JWA defines the algorithms used in JOSE and the JWK describes the handling of the cryptographic keys used in the process.
Often the term JWT is used when describing some other JOSE definitions so, for simplicity, in this article I will be using the term JWT.
JWT and REST
JWT have become very popular with the wide adoption of REST architectural style, but we can use JWT tokens to authenticate in various context, not only REST applications.
Tokens (and JWTs) are merely an authentication representations and so they can be used in multiple scenarios:
- REST services authentication
- OAuth 2.0 communications
- CSRF (Cross Site Request Forgery) protection schemes
- More in general as session IDs (eventually inside a cookie)
In this article(s) I will focus on REST technologies but I will give some example of using JWT in other contexts.
JWT benefits in a RESTful service
In his famous dissertation, Roy T. Fielding defines 6 constraints for (truly) RESTful services:
- Client/server architecture
- Stateless communication
- Cache (on the client)
- Uniform Interface
- Layered system
- Code on-demand
The most important (to me) is the second (and consequently the third one) that states REST interactions between client and server must be stateless by nature.
That means that requests (from the client) must contain all of the information necessary to understand the request and so they cannot take advantage of any stored context on the server and this, unfortunately, includes the session table typically stored on servers (automatic session management is one of the most publicized features of HTTP server frameworks).
The second constraint (if satisfied) induces the properties of visibility (looking at a request is sufficient to visualize the interaction), reliability (failure of one request does not influence others), and scalability (a server can switch a request to another server). Given these advantages you can see why this constraint is so important when building a REST server! Oh, and remember that JWT helps you to achieve this goal because:
- Using JWTs there’s no need of sessions
- Using JWTs there’s no need of session storage (on server)
- Using JWTs there’s no need of garbage collection of expired sessions
Conclusion
So, as you can see, JWT is a simple and yet powerful technology to accomplish several tasks.
In the next post I will explain in detail the JWT’s claims and we'll start to explore the delphi-jose-jwt library features.